Etsy API
How to use the Etsy API by using the OpenAPI spec to generate code for Java Spring Boot.
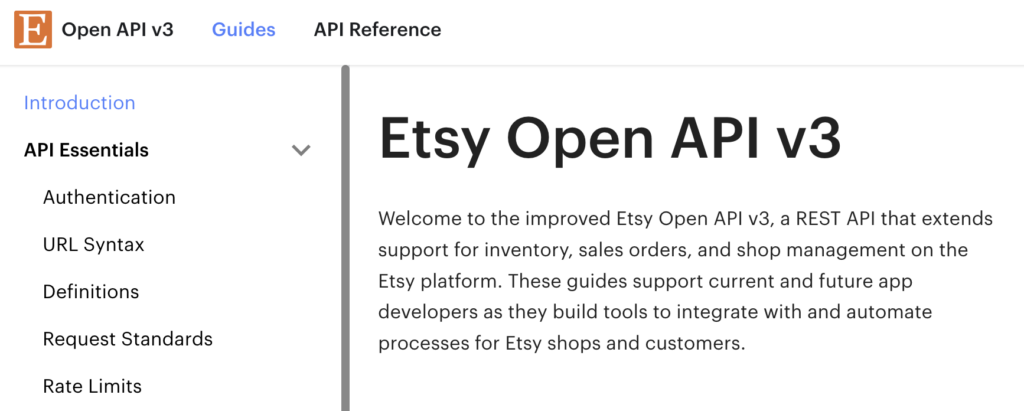
Introduction
During Covid, I setup an Esty store to sell custom 3d printed labels, I was manually setting the colour and text for each one.
Seems that I had found a niche and was selling labels, but after the 50th label I realized that I needed a way to automate the process.
Approach and Assumptions
The approach to automating the process was to use the Etsy API, OpenAPI Generater and Java.
The following are assumptions and dependencies:
- Etsy API 3, release 1.0.0
- OpenAPI Generator, openapi-generator-cli-6.2.0.jar
- Java 8
- MacOS, 12.6 (Monterey)
The Etsy API
Etsy provides an API to most of their functions, using the OpenAPI specification. Formerly known as Swagger, the OpenAPI provides definition of the RESTful web services in a json or yaml file.
The OpenAPI specification allows the API provider to share a language independent document of the API functionality. The clients using the API can also use the specification document to generate code that accesses the API.
Generating Java Code
“Use what you know” is a common refrain, so I used Java and SpringBoot to create a Java project that hosted the generated code based on the OpenAPI definition.
If you are looking for a Java client lib for Etsy, the generated Java that I created is available here:
https://github.com/gordonturner/etsy-open-api-client
This Java client lib was created from version 1.0.0 of the Etsy openapi.json file.
Download the OpenAPI Generator from:
https://repo1.maven.org/maven2/org/openapitools/openapi-generator-cli/6.2.0/openapi-generator-cli-6.2.0.jar
This assumes you have at least Java 8.0 installed, for more details see:
https://openapi-generator.tech/docs/installation/#jar
To generate the code, run the following command:
java -jar openapi-generator-cli-6.2.0.jar generate \
-i https://www.etsy.com/openapi/generated/oas/3.0.0.json \
-g java \
-o ./etsy-open-api-client \
--skip-validate-spec \
--group-id com.gordonturner.etsy \
--artifact-id etsy-open-api-client \
--artifact-version 1.1.0 \
--type-mappings=integer=Long,int=Long
It uses the OpenAPI spec file at https://www.etsy.com/openapi/generated/oas/3.0.0.json
Changes: Integers to Longs
Perhaps inevitably there are some manual changes and tweaks needed, often because the spec file was generated from one language and you are using it to generate code in another.
One of the first things I had to update was the size of some of the numeric elements. They were defined as Integers but the data returned from the API would not fit in the Java Integer object, so I had to add the type-mappings parameter in the generate command above.
This changed variables such as shopId, paymentIds, receiptId etc.
Changes: Enums
The next change was that the values returned from the Etsy API for org.openapitools.client.model.ShopReceipt.StatusEnum
where mixed case values, and the generated definition expected all lower case.
I opened a GitHub issue (https://github.com/etsy/open-api/issues/469), but in the mean time I updated the generated Enum file to expect mixed case.
What functionality does the Etsy API have?
All this is great, but what can I do with it?
Well, I used it to create a private website that lists all of the open orders and then added a button to create an order directory and add a data.yaml
file describing each order’s label (model, label text and colours).
Then I can then read in that data in Fusion 360, to automate the creation of the printer stl files.
Additionally, I can also see what the actual revenue from an order is, excluding the Etsy and shipping fees.
Conclusion
Prior to OpenAPI specification, the consumption of API services was a real chore. Now the effort to keep things (mostly) up to date is much lower and enables custom solutions to stream line workflows.